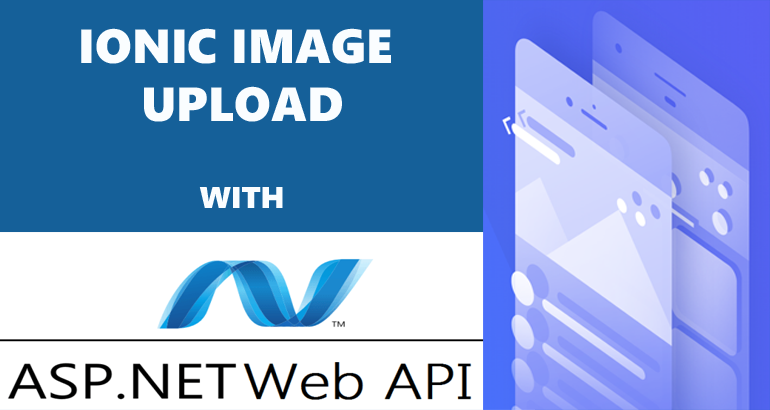
Many .Net developers have struggled so much to upload image from an Ionic application with ASP.Net Web API.
In this tutorial, I will show you how easy it is to upload image in an ionic application via .Net Web API.
Since this tutorial is not for beginner I will skip how to create a new ionic app via ionic CLI.
ASP.Net Web API Controller
Below is the web api controller that will handle the image upload:[HttpPost] [Route("upload")] public HttpResponseMessage uploadImage() { var request = HttpContext.Current.Request; if (Request.Content.IsMimeMultipartContent()) { if (request.Files.Count > 0) { var postedFile = request.Files.Get("file"); var title = request.Params["title"]; string root = HttpContext.Current.Server.MapPath("~/Images"); root = root + "/" + postedFile.FileName; postedFile.SaveAs(root); //Save post to DB return Request.CreateResponse(HttpStatusCode.Found, new { error = false, status = "created", path = root }); } } return null; }
The above code is self explanatory, however I want you to take note of these two lines:
var postedFile = request.Files.Get("file"); var title = request.Params["title"];
I will be making reference to those two line in the ionic app code.
Setting up the ionic project
Create a blank ionic app and install the following cordova and ionic-native plugins:ionic cordova plugin add cordova-plugin-file npm install --save @ionic-native/file ionic cordova plugin add cordova-plugin-camera npm install --save @ionic-native/camera ionic cordova plugin add cordova-plugin-file-transfer npm install --save @ionic-native/file-transfer
Open up the app.module.ts file - 'src/app/app.module.ts' and all needed imports and also include them in the providers section of the NgModule.
import { FileTransfer, FileUploadOptions, FileTransferObject } from '@ionic-native/file-transfer'; import { File } from '@ionic-native/file'; import { Camera } from '@ionic-native/camera'; providers: [ StatusBar, SplashScreen, {provide: ErrorHandler, useClass: IonicErrorHandler}, FileTransfer, FileTransferObject, File, Camera ]
Modify the home.html page to look like so:
That just create a form that has a title field and image field. I am also using Ng-If-Else to control which component to show when an image has been selected or not. Fairly simple.
Add the simple css to home.scss file to make the screen look good
ion-card{ margin: 3px !important; width: calc(100% - 6px) !important; .uploadWrap{ ion-icon{ color:#777; font-size: 150px; text-align: center !important; display: block; } } button{ height: 40px !important; } }
Let's move to the home.ts file where we will be doing the major work. Add the required imports
import { FileTransfer, FileTransferObject, FileUploadOptions } from '@ionic-native/file-transfer'; import { Camera, CameraOptions } from '@ionic-native/camera';
Declare variables needed:
imageURI: any; imageTitle: any; isImageSelected:boolean = false;
In this app we will just be getting image from gallery. Add the following code that allow us choose image from the device gallery:
chooseFile() { let options: CameraOptions = { quality: 100, destinationType: this.camera.DestinationType.FILE_URI, sourceType: this.camera.PictureSourceType.PHOTOLIBRARY, allowEdit: true, encodingType: this.camera.EncodingType.JPEG, targetWidth: 500, targetHeight: 500, saveToPhotoAlbum: false }; this.camera.getPicture(options).then((img) => { this.imageURI = img; this.isImageSelected =true; }).catch((reason) => { console.log(reason); }); }
The full home.ts file should now look like this
import { Component } from '@angular/core'; import { IonicPage, NavController, NavParams, LoadingController } from 'ionic-angular'; import { FileTransfer, FileTransferObject, FileUploadOptions } from '@ionic-native/file-transfer'; import { Camera, CameraOptions } from '@ionic-native/camera'; @IonicPage() @Component({ selector: 'page-home', templateUrl: 'home.html', }) export class HomePage { imageURI: any; imageTitle: any; isImageSelected:boolean = false; constructor(public navCtrl: NavController, public navParams: NavParams, private transfer: FileTransfer, private camera: Camera, public loadingCtrl: LoadingController) { } chooseFile() { let options: CameraOptions = { quality: 100, destinationType: this.camera.DestinationType.FILE_URI, sourceType: this.camera.PictureSourceType.PHOTOLIBRARY, allowEdit: true, encodingType: this.camera.EncodingType.JPEG, targetWidth: 500, targetHeight: 500, saveToPhotoAlbum: false }; this.camera.getPicture(options).then((img) => { this.imageURI = img; this.isImageSelected =true; }).catch((reason) => { console.log(reason); }); } doImageUpload() { let loader = this.loadingCtrl.create({ content: "Uploading..." }); loader.present(); let filename = this.imageURI.split('/').pop(); const fileTransfer: FileTransferObject = this.transfer.create(); let options: FileUploadOptions = { fileKey: "file", fileName: filename, chunkedMode: false, mimeType: "image/jpg", params: { 'title': this.imageTitle } }; fileTransfer.upload(this.imageURI, "http://localhost/api/upload",options).then((res)=>{ },(err)=>{ }); } }In the doImageUpload function above there two things that are important to these two lines I talked about in the web api controller
var postedFile = request.Files.Get("file"); var title = request.Params["title"];
fileKey: "file"
params: { 'title': this.imageTitle }
The file and the title in the params object. You can add more parameters to the params object and get the value with they key in the api.
Happy coding!
Post a Comment
0Comments